Embedthis ESP™
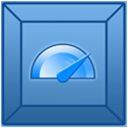
ESP is archived and no longer supported or maintained.
ESP is a web framework that makes it dramatically easier to create blazing fast, dynamic embedded web applications and web sites. ESP applications use the C language for server-side programming, which allows easy access to system or hardware data. The result is fast and responsive web applications.
ESP is not a traditional low-level environment. It is a powerhouse MVC framework in a tiny footprint. ESP has all the features you would normally expect from an enterprise web framework. ESP is also great for development and for creating single page applications (SPAs). If web pages or controllers are modified, the code is transparently recompiled and reloaded. This enables unparalleled performance with "script-like" flexibility for web applications.
ESP Components
The ESP web framework provides a complete set of components including
- HTTP web server with HTTP/2 support
- Model-View-Controller framework
- Dynamic loader for C controllers
- Templating with embedded C code in web pages
- Application and scaffold generator
- Web Sockets
- Embedded databases: SQLite and In-memory DB
- Database migrations
- Extensive programmer API
- Library of extension packages
- Skeletons and generators to get going quickly
- Complete tooling for deploying web applications
This document describes the ESP web framework and how to use ESP.
ESP Patterns
ESP supports several design patterns:
- Stand-alone ESP web pages
- Single Page Applications (SPA) using VueJS or other Javascript client frameworks.
- ESP Model-View-Controller applications (MVC)
- Web Services via ESP Controllers
- Web Sockets live management applications
Your applications can choose a predominating pattern or you can mix patterns in a single application if desired.
Stand-alone Pages
An ESP page is a standard HTML web page with embedded C code that executes at run-time to create a dynamic client response. Pages typically have a ".esp" extension and may use ESP layout pages to define the look and feel of the application in one place.
The C code is embedded between <% and %> tags. For example:
<h1>Hello World</h1> <p><% render("A random number %d", rand(0)); %></p>
When the web page is requested, the web page including the code inside the ESP tags is compiled and saved as a shared library. When the page is requested, the call to render will replace the code between <% ... %> with the result of the render call. Finally, then the composite page will be sent to the client. The next time the page is requested, it will be transparently recompiled and reloaded if the source web page has been modified See ESP Pages below for more details.
Web Services
ESP is especially well suited for high performance web services. ESP permits the binding of URLs directly to C functions. When the URL is requested, the corresponding C function, typically called an action, is executed to dynamically generate a response. The actions are typically grouped into a loadable ESP controller file. For example:
/* Hello Action */ static void hello() { render("{greeting: 'Hello World'}\n"); finalize(); } /* Initialization of Actions */ ESP_EXPORT int esp_module_greeting(HttpRoute *route) { espAction(route, "greeting-hello", "user", hello); return 0; }
This example is a loadable ESP controller with one action function. The actions are published by binding functions to route names. When the appropriate URL is requested, the hello function is run to generate the response. During development, if the file containing this code is modified, the page will be transparently recompiled and reloaded before running the new code.
Model-View-Controller
A Model-View Controller application, also known as an MVC app, is a proven paradigm for organizing larger web applications. In this pattern, it is the "model" that manages the state of the application including the database. The "controller" responds to inputs and invokes then the relevant "views" to generate the user interface. Originally developed in the '70s, it has been more recently adapted for web applications and been popularized by frameworks such as Ruby on Rails. ESP uses the same paradigm with an embedded spin.
ESP supports MVC applications and provides an esp application generator to create MVC applications and scaffolds. ESP support two styles of MVC applications:
- Single Page Applications
- Server-side MVC Applications
Server-side MVC Applications
ESP implements server-side MVC applications by providing using the Expansive web site generator. Expansive provides development time scripting, layout pages, partial pages to support the easy insertion of dynamic data into web pages before they are delivered to the client. A common look-and-feel is achieved by using master layout pages.
Single Page Applications
Single page applications deliver the entire web application as a single page and then incrementally download resources and data in the background as required. With this paradigm, it is the client that defines the entire user interface via a client-side Javascript framework such as VueJS. The server provides the initial page load and ongoing data. This approach can provide a more responsive and fluid user experience without the delays caused by page reloads. ESP is well suited to SPA applications and can deliver all the required resources and live data for such apps.
ESP Web Framework
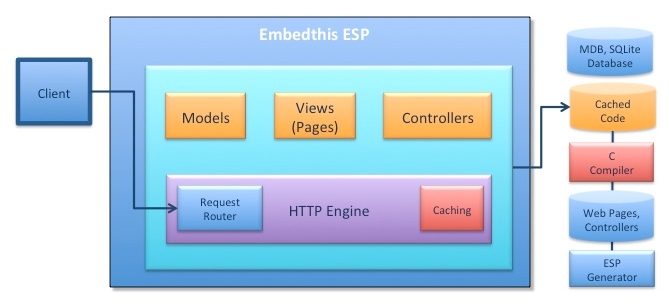
The main components of the ESP Web Framework are:
- HTTP Web Server
- ESP Pages
- ESP Controllers
- ESP Models
- ESP Compilation
- ESP Generator
- ESP API
HTTP Web Server
ESP includes the Embedthis HTTP engine that has been used for years in Appweb. This is a high performance, event-based, multithreaded HTTP engine. It fully supports HTTP/1.1 with extensions for WebSockets, Sessions, SSL, Security Limits and Defensive counter-measures.
ESP Pages
ESP Pages provide the "View" portion of MVC frameworks and are responsible for generating the user interface. They provide:
- Embedded C language code for dynamic HTML responses
- An ESP API library of convenience routines to manage web requests, session state and render output
Note that if using ESP for a Single Page Architecture application, the views are typically generated by the client using a client-side framework such as Aurelia. In this case, ESP views are used for the initial, single home page.
ESP Page Directives
ESP supports the following special sequences for use in web pages.
Directive | Purpose |
---|---|
<% code %> | Insert code at this location. At run-time, this C code will execute. |
<%= code %> | Evaluate the expression and substitute the resulting value into the web page at this position. A printf format specifier can be used to specify a different variable type. For example, <%= %d ivar %> C code will execute. |
%!variable | Shortcut for <%= variable %> |
%!{expression} | Shortcut for <%= expression %> |
%$param | Substitutes the value of a request parameter or session variable of the same name after HTML escaping the value. |
%#field | Substitute the field name in the current database record. |
%~ | Insert a URL to the top of the application. |
See ESP Page Directives for more details.
ESP Controllers
ESP Controllers are collections of C functions that are bound to specific URLs for the purpose of managing a resource or group of resources. Often a controller will use RESTful routes to map URLs to specific actions.
A controller is typically coded in a single source file, though it can reference any external libraries. The controller will be dynamically compiled, saved and loaded when a request is received for any action in the controller. If the source code is modified, the controller will be recompiled and reloaded. Alternatively, the esp command can be used to precompile controllers so that a compiler is not required on the target system in production. Compiled controllers are saved in a cache directory as shared libraries.
Actions
When ESP receives a request to service, the request is parsed and then dispatched to a specific function in the controller called an action. The job of the action function is to:
- Respond to the request
- Render a response view back to the client
How the action responds to the request depends on the request parameters, the query information, form parameters and other HTTP and application state information.
Here is a sample action function that updates a database table based on user submitted form data in "params".
static void update() { if (updateFields("post", params())) { inform("Post updated successfully."); redirect("list"); } else { renderView("post-edit"); } }
An action function can explicitly render data by calling one of the render methods. It can redirect the client to a new URI via the redirect method.
If the action method does not explicitly render any data, ESP will render an ESP web page of the same name as the action function. That web page has full access to the controller and request state. This is a common pattern where a controller will respond to a request and an ESP view page will format the response to the client.
ESP Models and Databases
The Model part of an MVC framework stores the application data and access logic and may provide an Object Relational Mapping (ORM) layer. Unfortunately, while being powerful and extensive, ORMs often consume considerable amounts of memory and can be slow at run-time. ESP avoids this problem by providing a simple mapping from relational database data to C based records and grids. This results in a high-performance database interface with very low memory requirements.
Embedded Database Interface
ESP supports an Embedded Database Interface (EDI) above a user-selectable database such as: SQLite or MDB. The MDB database is a tiny, in-memory database — great for embedded applications. SQLite is a full SQL implementation for embedded applications. MDB is the smallest and fastest, but has the least features.
Migrations
The ESP generator program can create database migration modules. These are mini-programs that create and modify database tables. Migrations are useful to create databases and test data.
ESP API
The ESP web framework has an extensive suite of APIs to cover all possible design needs. It also defines an expressive, terse, short-form API that makes common tasks simple. See the ESP API Reference for more details.
ESP uses a garbage collector so that you never need to free ESP memory allocated in web pages or in controllers. This results in more secure, reliable web applications.